Avatar
Avatars can be used to represent people or objects. It supports images, Icon
s, or letters.
Examples
TypeScript
JavaScript
import { UserOutlined } from '@ant-design/icons';
import { Avatar } from 'antd';
import React from 'react';
const App: React.FC = () => (
<>
<div>
<Avatar size={64} icon={<UserOutlined />} />
<Avatar size="large" icon={<UserOutlined />} />
<Avatar icon={<UserOutlined />} />
<Avatar size="small" icon={<UserOutlined />} />
</div>
<div>
<Avatar shape="square" size={64} icon={<UserOutlined />} />
<Avatar shape="square" size="large" icon={<UserOutlined />} />
<Avatar shape="square" icon={<UserOutlined />} />
<Avatar shape="square" size="small" icon={<UserOutlined />} />
</div>
</>
);
export default App;
TypeScript
JavaScript
import { Avatar, Button } from 'antd';
import React, { useState } from 'react';
const UserList = ['U', 'Lucy', 'Tom', 'Edward'];
const ColorList = ['#f56a00', '#7265e6', '#ffbf00', '#00a2ae'];
const GapList = [4, 3, 2, 1];
const App: React.FC = () => {
const [user, setUser] = useState(UserList[0]);
const [color, setColor] = useState(ColorList[0]);
const [gap, setGap] = useState(GapList[0]);
const changeUser = () => {
const index = UserList.indexOf(user);
setUser(index < UserList.length - 1 ? UserList[index + 1] : UserList[0]);
setColor(index < ColorList.length - 1 ? ColorList[index + 1] : ColorList[0]);
};
const changeGap = () => {
const index = GapList.indexOf(gap);
setGap(index < GapList.length - 1 ? GapList[index + 1] : GapList[0]);
};
return (
<>
<Avatar style={{ backgroundColor: color, verticalAlign: 'middle' }} size="large" gap={gap}>
{user}
</Avatar>
<Button
size="small"
style={{ margin: '0 16px', verticalAlign: 'middle' }}
onClick={changeUser}
>
ChangeUser
</Button>
<Button size="small" style={{ verticalAlign: 'middle' }} onClick={changeGap}>
changeGap
</Button>
</>
);
};
export default App;
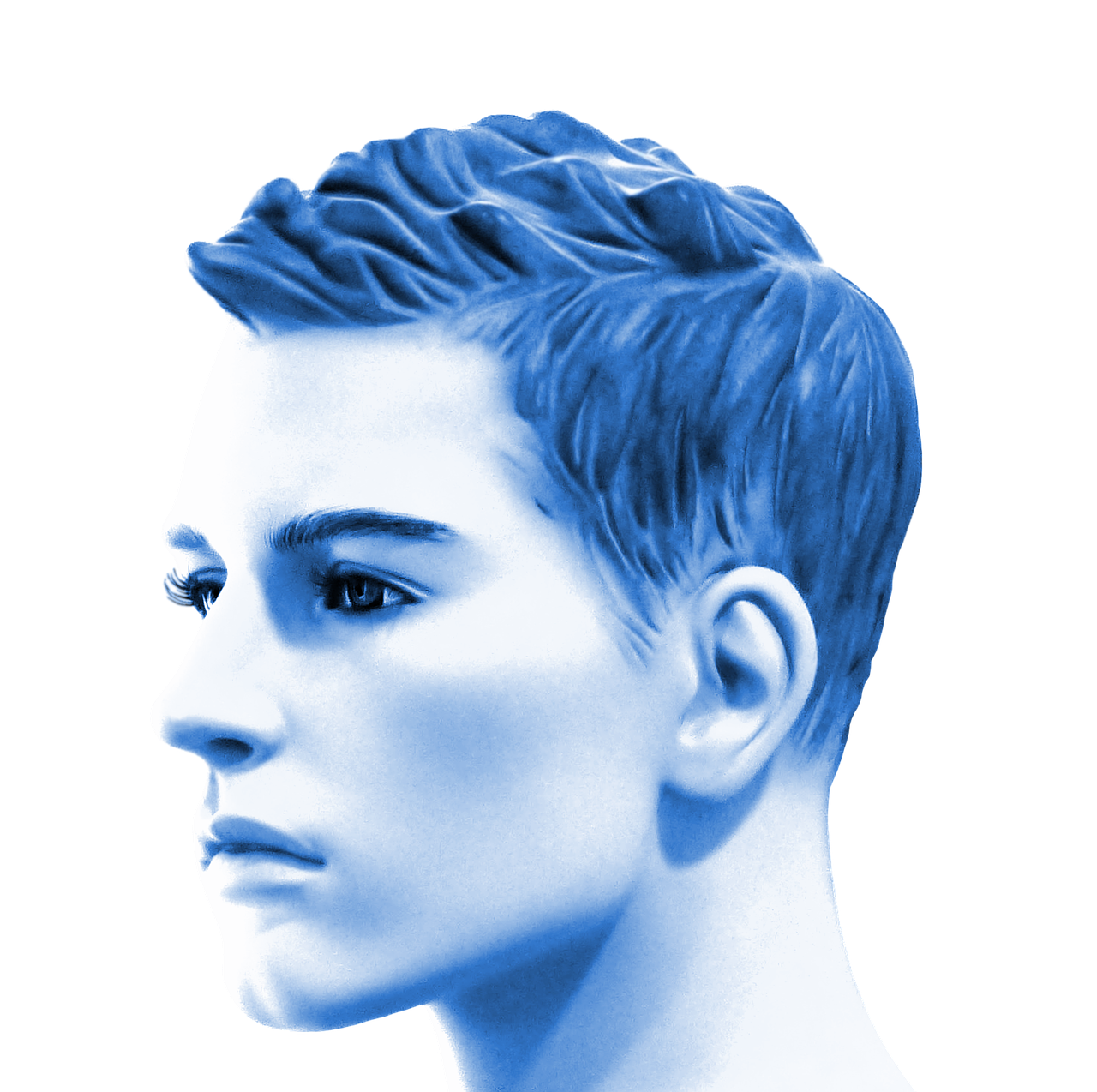
TypeScript
JavaScript
import { AntDesignOutlined, UserOutlined } from '@ant-design/icons';
import { Avatar, Divider, Tooltip } from 'antd';
import React from 'react';
const App: React.FC = () => (
<>
<Avatar.Group>
<Avatar src="https://joeschmoe.io/api/v1/random" />
<Avatar style={{ backgroundColor: '#f56a00' }}>K</Avatar>
<Tooltip title="Ant User" placement="top">
<Avatar style={{ backgroundColor: '#87d068' }} icon={<UserOutlined />} />
</Tooltip>
<Avatar style={{ backgroundColor: '#1890ff' }} icon={<AntDesignOutlined />} />
</Avatar.Group>
<Divider />
<Avatar.Group maxCount={2} maxStyle={{ color: '#f56a00', backgroundColor: '#fde3cf' }}>
<Avatar src="https://joeschmoe.io/api/v1/random" />
<Avatar style={{ backgroundColor: '#f56a00' }}>K</Avatar>
<Tooltip title="Ant User" placement="top">
<Avatar style={{ backgroundColor: '#87d068' }} icon={<UserOutlined />} />
</Tooltip>
<Avatar style={{ backgroundColor: '#1890ff' }} icon={<AntDesignOutlined />} />
</Avatar.Group>
<Divider />
<Avatar.Group
maxCount={2}
size="large"
maxStyle={{ color: '#f56a00', backgroundColor: '#fde3cf' }}
>
<Avatar src="https://joeschmoe.io/api/v1/random" />
<Avatar style={{ backgroundColor: '#f56a00' }}>K</Avatar>
<Tooltip title="Ant User" placement="top">
<Avatar style={{ backgroundColor: '#87d068' }} icon={<UserOutlined />} />
</Tooltip>
<Avatar style={{ backgroundColor: '#1890ff' }} icon={<AntDesignOutlined />} />
</Avatar.Group>
<Divider />
<Avatar.Group
maxCount={2}
maxPopoverTrigger="click"
size="large"
maxStyle={{ color: '#f56a00', backgroundColor: '#fde3cf', cursor: 'pointer' }}
>
<Avatar src="https://zos.alipayobjects.com/rmsportal/ODTLcjxAfvqbxHnVXCYX.png" />
<Avatar style={{ backgroundColor: '#f56a00' }}>K</Avatar>
<Tooltip title="Ant User" placement="top">
<Avatar style={{ backgroundColor: '#87d068' }} icon={<UserOutlined />} />
</Tooltip>
<Avatar style={{ backgroundColor: '#1890ff' }} icon={<AntDesignOutlined />} />
</Avatar.Group>
</>
);
export default App;
Preview
TypeScript
JavaScript
import { UserOutlined } from '@ant-design/icons';
import { Avatar, Image } from 'antd';
import React from 'react';
const App: React.FC = () => (
<>
<Avatar icon={<UserOutlined />} />
<Avatar>U</Avatar>
<Avatar size={40}>USER</Avatar>
<Avatar src="https://joeschmoe.io/api/v1/random" />
<Avatar src={<Image src="https://joeschmoe.io/api/v1/random" style={{ width: 32 }} />} />
<Avatar style={{ color: '#f56a00', backgroundColor: '#fde3cf' }}>U</Avatar>
<Avatar style={{ backgroundColor: '#87d068' }} icon={<UserOutlined />} />
</>
);
export default App;
TypeScript
JavaScript
import { UserOutlined } from '@ant-design/icons';
import { Avatar, Badge } from 'antd';
import React from 'react';
const App: React.FC = () => (
<>
<span className="avatar-item">
<Badge count={1}>
<Avatar shape="square" icon={<UserOutlined />} />
</Badge>
</span>
<span>
<Badge dot>
<Avatar shape="square" icon={<UserOutlined />} />
</Badge>
</span>
</>
);
export default App;
/* tile uploaded pictures */
.avatar-item {
margin-right: 24px;
}
[class*='-col-rtl'] .avatar-item {
margin-right: 0;
margin-left: 24px;
}
TypeScript
JavaScript
import { AntDesignOutlined } from '@ant-design/icons';
import { Avatar } from 'antd';
import React from 'react';
const App: React.FC = () => (
<Avatar
size={{ xs: 24, sm: 32, md: 40, lg: 64, xl: 80, xxl: 100 }}
icon={<AntDesignOutlined />}
/>
);
export default App;
API#
Avatar#
Property | Description | Type | Default | Version |
---|---|---|---|---|
alt | This attribute defines the alternative text describing the image | string | - | |
gap | Letter type unit distance between left and right sides | number | 4 | 4.3.0 |
icon | Custom icon type for an icon avatar | ReactNode | - | |
shape | The shape of avatar | circle | square | circle | |
size | The size of the avatar | number | large | small | default | { xs: number, sm: number, ...} | default | 4.7.0 |
src | The address of the image for an image avatar or image element | string | ReactNode | - | ReactNode: 4.8.0 |
srcSet | A list of sources to use for different screen resolutions | string | - | |
draggable | Whether the picture is allowed to be dragged | boolean | 'true' | 'false' | - | |
crossOrigin | CORS settings attributes | 'anonymous' | 'use-credentials' | '' | - | 4.17.0 |
onError | Handler when img load error, return false to prevent default fallback behavior | () => boolean | - |
Tip: You can set
icon
orchildren
as the fallback for image load error, with the priority oficon
>children
Avatar.Group (4.5.0+)#
Property | Description | Type | Default | Version |
---|---|---|---|---|
maxCount | Max avatars to show | number | - | |
maxPopoverPlacement | The placement of excess avatar Popover | top | bottom | top | |
maxPopoverTrigger | Set the trigger of excess avatar Popover | hover | focus | click | hover | 4.17.0 |
maxStyle | The style of excess avatar style | CSSProperties | - | |
size | The size of the avatar | number | large | small | default | { xs: number, sm: number, ...} | default | 4.8.0 |