Card
Simple rectangular container.
When To Use#
A card can be used to display content related to a single subject. The content can consist of multiple elements of varying types and sizes.
Examples
Default size card
Card content
Card content
Card content
Small size card
Card content
Card content
Card content
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const App: React.FC = () => (
<>
<Card title="Default size card" extra={<a href="#">More</a>} style={{ width: 300 }}>
<p>Card content</p>
<p>Card content</p>
<p>Card content</p>
</Card>
<Card size="small" title="Small size card" extra={<a href="#">More</a>} style={{ width: 300 }}>
<p>Card content</p>
<p>Card content</p>
<p>Card content</p>
</Card>
</>
);
export default App;
Card title
Card content
Card content
Card content
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const App: React.FC = () => (
<div className="site-card-border-less-wrapper">
<Card title="Card title" bordered={false} style={{ width: 300 }}>
<p>Card content</p>
<p>Card content</p>
<p>Card content</p>
</Card>
</div>
);
export default App;
.site-card-border-less-wrapper {
padding: 30px;
background: #ececec;
}
Card content
Card content
Card content
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const App: React.FC = () => (
<Card style={{ width: 300 }}>
<p>Card content</p>
<p>Card content</p>
<p>Card content</p>
</Card>
);
export default App;
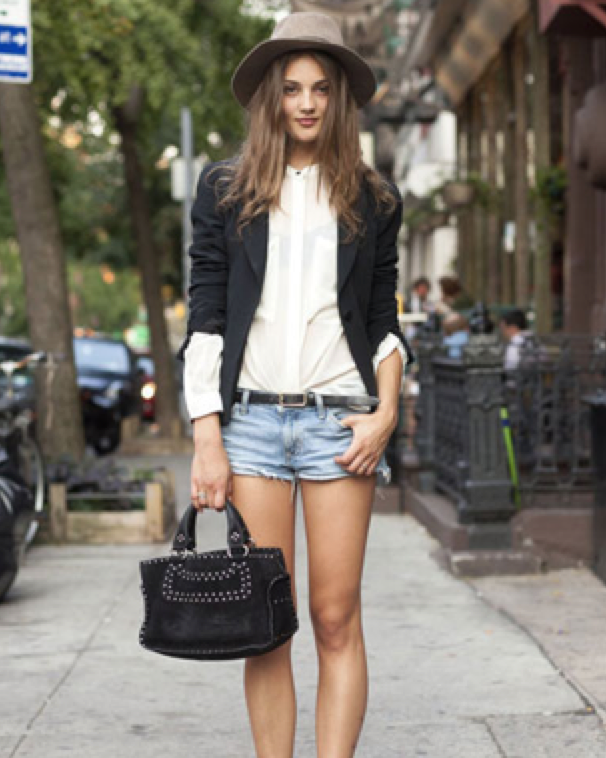
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const { Meta } = Card;
const App: React.FC = () => (
<Card
hoverable
style={{ width: 240 }}
cover={<img alt="example" src="https://os.alipayobjects.com/rmsportal/QBnOOoLaAfKPirc.png" />}
>
<Meta title="Europe Street beat" description="www.instagram.com" />
</Card>
);
export default App;
Card title
Card content
Card title
Card content
Card title
Card content
TypeScript
JavaScript
import { Card, Col, Row } from 'antd';
import React from 'react';
const App: React.FC = () => (
<div className="site-card-wrapper">
<Row gutter={16}>
<Col span={8}>
<Card title="Card title" bordered={false}>
Card content
</Card>
</Col>
<Col span={8}>
<Card title="Card title" bordered={false}>
Card content
</Card>
</Col>
<Col span={8}>
<Card title="Card title" bordered={false}>
Card content
</Card>
</Col>
</Row>
</div>
);
export default App;
TypeScript
JavaScript
import { EditOutlined, EllipsisOutlined, SettingOutlined } from '@ant-design/icons';
import { Avatar, Card, Skeleton, Switch } from 'antd';
import React, { useState } from 'react';
const { Meta } = Card;
const App: React.FC = () => {
const [loading, setLoading] = useState(true);
const onChange = (checked: boolean) => {
setLoading(!checked);
};
return (
<>
<Switch checked={!loading} onChange={onChange} />
<Card style={{ width: 300, marginTop: 16 }} loading={loading}>
<Meta
avatar={<Avatar src="https://joeschmoe.io/api/v1/random" />}
title="Card title"
description="This is the description"
/>
</Card>
<Card
style={{ width: 300, marginTop: 16 }}
actions={[
<SettingOutlined key="setting" />,
<EditOutlined key="edit" />,
<EllipsisOutlined key="ellipsis" />,
]}
>
<Skeleton loading={loading} avatar active>
<Meta
avatar={<Avatar src="https://joeschmoe.io/api/v1/random" />}
title="Card title"
description="This is the description"
/>
</Skeleton>
</Card>
</>
);
};
export default App;
Card Title
Content
Content
Content
Content
Content
Content
Content
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const gridStyle: React.CSSProperties = {
width: '25%',
textAlign: 'center',
};
const App: React.FC = () => (
<Card title="Card Title">
<Card.Grid style={gridStyle}>Content</Card.Grid>
<Card.Grid hoverable={false} style={gridStyle}>
Content
</Card.Grid>
<Card.Grid style={gridStyle}>Content</Card.Grid>
<Card.Grid style={gridStyle}>Content</Card.Grid>
<Card.Grid style={gridStyle}>Content</Card.Grid>
<Card.Grid style={gridStyle}>Content</Card.Grid>
<Card.Grid style={gridStyle}>Content</Card.Grid>
</Card>
);
export default App;
TypeScript
JavaScript
import { Card } from 'antd';
import React from 'react';
const App: React.FC = () => (
<Card title="Card title">
<Card type="inner" title="Inner Card title" extra={<a href="#">More</a>}>
Inner Card content
</Card>
<Card
style={{ marginTop: 16 }}
type="inner"
title="Inner Card title"
extra={<a href="#">More</a>}
>
Inner Card content
</Card>
</Card>
);
export default App;
Card title
tab1
tab2
content1
article
app
project
app content
TypeScript
JavaScript
import { Card } from 'antd';
import React, { useState } from 'react';
const tabList = [
{
key: 'tab1',
tab: 'tab1',
},
{
key: 'tab2',
tab: 'tab2',
},
];
const contentList: Record<string, React.ReactNode> = {
tab1: <p>content1</p>,
tab2: <p>content2</p>,
};
const tabListNoTitle = [
{
key: 'article',
tab: 'article',
},
{
key: 'app',
tab: 'app',
},
{
key: 'project',
tab: 'project',
},
];
const contentListNoTitle: Record<string, React.ReactNode> = {
article: <p>article content</p>,
app: <p>app content</p>,
project: <p>project content</p>,
};
const App: React.FC = () => {
const [activeTabKey1, setActiveTabKey1] = useState<string>('tab1');
const [activeTabKey2, setActiveTabKey2] = useState<string>('app');
const onTab1Change = (key: string) => {
setActiveTabKey1(key);
};
const onTab2Change = (key: string) => {
setActiveTabKey2(key);
};
return (
<>
<Card
style={{ width: '100%' }}
title="Card title"
extra={<a href="#">More</a>}
tabList={tabList}
activeTabKey={activeTabKey1}
onTabChange={key => {
onTab1Change(key);
}}
>
{contentList[activeTabKey1]}
</Card>
<br />
<br />
<Card
style={{ width: '100%' }}
tabList={tabListNoTitle}
activeTabKey={activeTabKey2}
tabBarExtraContent={<a href="#">More</a>}
onTabChange={key => {
onTab2Change(key);
}}
>
{contentListNoTitle[activeTabKey2]}
</Card>
</>
);
};
export default App;

TypeScript
JavaScript
import { EditOutlined, EllipsisOutlined, SettingOutlined } from '@ant-design/icons';
import { Avatar, Card } from 'antd';
import React from 'react';
const { Meta } = Card;
const App: React.FC = () => (
<Card
style={{ width: 300 }}
cover={
<img
alt="example"
src="https://gw.alipayobjects.com/zos/rmsportal/JiqGstEfoWAOHiTxclqi.png"
/>
}
actions={[
<SettingOutlined key="setting" />,
<EditOutlined key="edit" />,
<EllipsisOutlined key="ellipsis" />,
]}
>
<Meta
avatar={<Avatar src="https://joeschmoe.io/api/v1/random" />}
title="Card title"
description="This is the description"
/>
</Card>
);
export default App;
API#
<Card title="Card title">Card content</Card>
Card#
Property | Description | Type | Default | Version |
---|---|---|---|---|
actions | The action list, shows at the bottom of the Card | Array<ReactNode> | - | |
activeTabKey | Current TabPane's key | string | - | |
bodyStyle | Inline style to apply to the card content | CSSProperties | - | |
bordered | Toggles rendering of the border around the card | boolean | true | |
cover | Card cover | ReactNode | - | |
defaultActiveTabKey | Initial active TabPane's key, if activeTabKey is not set | string | - | |
extra | Content to render in the top-right corner of the card | ReactNode | - | |
headStyle | Inline style to apply to the card head | CSSProperties | - | |
hoverable | Lift up when hovering card | boolean | false | |
loading | Shows a loading indicator while the contents of the card are being fetched | boolean | false | |
size | Size of card | default | small | default | |
tabBarExtraContent | Extra content in tab bar | ReactNode | - | |
tabList | List of TabPane's head | Array<{key: string, tab: ReactNode}> | - | |
tabProps | Tabs | - | - | |
title | Card title | ReactNode | - | |
type | Card style type, can be set to inner or not set | string | - | |
onTabChange | Callback when tab is switched | (key) => void | - |
Card.Grid#
Property | Description | Type | Default | Version |
---|---|---|---|---|
className | The className of container | string | - | |
hoverable | Lift up when hovering card grid | boolean | true | |
style | The style object of container | CSSProperties | - |
Card.Meta#
Property | Description | Type | Default | Version |
---|---|---|---|---|
avatar | Avatar or icon | ReactNode | - | |
className | The className of container | string | - | |
description | Description content | ReactNode | - | |
style | The style object of container | CSSProperties | - | |
title | Title content | ReactNode | - |